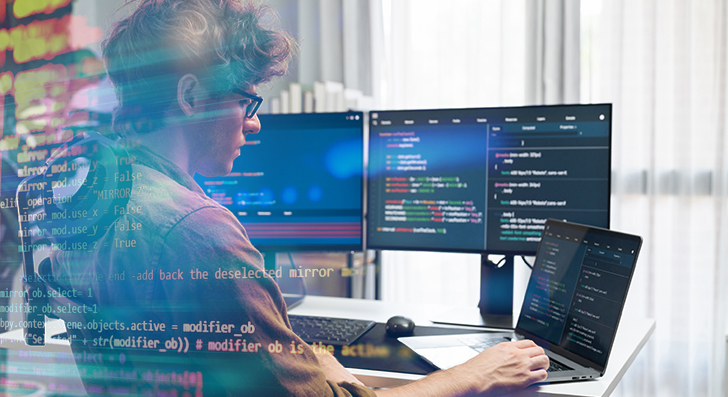
Scalability implies your application can manage growth—extra people, a lot more information, and much more traffic—without having breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't a thing you bolt on later—it ought to be component within your program from the beginning. Quite a few programs are unsuccessful whenever they grow rapidly because the initial design can’t handle the extra load. To be a developer, you should Assume early about how your technique will behave stressed.
Begin by designing your architecture being flexible. Stay clear of monolithic codebases the place every thing is tightly linked. Instead, use modular design and style or microservices. These styles break your application into smaller sized, impartial parts. Every single module or assistance can scale on its own devoid of influencing the whole method.
Also, contemplate your database from day a person. Will it want to manage a million consumers or merely a hundred? Pick the ideal kind—relational or NoSQL—determined by how your facts will mature. Strategy for sharding, indexing, and backups early, Even though you don’t will need them yet.
Yet another vital point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath present-day circumstances. Take into consideration what would take place When your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like concept queues or function-driven devices. These enable your application take care of far more requests with no receiving overloaded.
Any time you Make with scalability in your mind, you're not just making ready for fulfillment—you might be lessening upcoming problems. A very well-planned program is simpler to maintain, adapt, and mature. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a important part of developing scalable purposes. Not all databases are designed the identical, and using the Completely wrong one can slow you down or maybe lead to failures as your app grows.
Start out by being familiar with your knowledge. Is it remarkably structured, like rows within a table? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. They are solid with interactions, transactions, and consistency. In addition they help scaling techniques like examine replicas, indexing, and partitioning to deal with extra targeted traffic and information.
In case your facts is much more flexible—like consumer exercise logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured info and may scale horizontally additional effortlessly.
Also, look at your read and publish styles. Are you currently executing lots of reads with less writes? Use caching and browse replicas. Have you been managing a heavy publish load? Take a look at databases that will cope with high create throughput, as well as party-primarily based data storage techniques like Apache Kafka (for momentary data streams).
It’s also wise to Consider in advance. You might not need Innovative scaling options now, but deciding on a database that supports them suggests you received’t need to change later on.
Use indexing to speed up queries. Prevent pointless joins. Normalize or denormalize your info dependant upon your obtain styles. And usually keep an eye on databases effectiveness when you mature.
In short, the proper database is determined by your app’s construction, speed desires, And just how you be expecting it to improve. Acquire time to choose properly—it’ll preserve plenty of difficulty later.
Improve Code and Queries
Speedy code is essential to scalability. As your application grows, each individual smaller hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your procedure. That’s why it’s essential to Create productive logic from the beginning.
Commence by creating clean, uncomplicated code. Keep away from repeating logic and remove anything avoidable. Don’t select the most complicated solution if an easy a single operates. Keep your capabilities quick, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single question only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And prevent performing a lot of joins, especially throughout large tables.
In case you notice the identical facts becoming requested time and again, use caching. Store the outcome quickly utilizing instruments like Redis or Memcached and that means you don’t need to repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional efficient.
Remember to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when desired. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more end users plus much more targeted visitors. If every thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. These two applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across numerous servers. Rather than 1 server doing all the do the job, the load balancer routes people to diverse servers depending on availability. This means no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the others. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When consumers request the exact same data again—like an item webpage or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quickly obtain.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static files near to the person.
Caching lowers databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And always ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with more consumers, keep fast, and Recuperate from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to purchase hardware or guess potential capability. When site visitors click here will increase, it is possible to insert more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on building your application in place of taking care of infrastructure.
Containers are One more vital Resource. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it effortless to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If one section of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale sections independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get well swiftly when complications come about. If you want your app to mature without having restrictions, begin employing these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not fixing.
Watch Everything
Should you don’t watch your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot concerns early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it takes for users to load pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified quickly. This will help you deal with difficulties rapidly, usually just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to serious problems.
As your app grows, traffic and facts boost. With out monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you keep in control.
Briefly, monitoring allows you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for big corporations. Even little applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make applications that expand efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.